Tissot's indicatrix
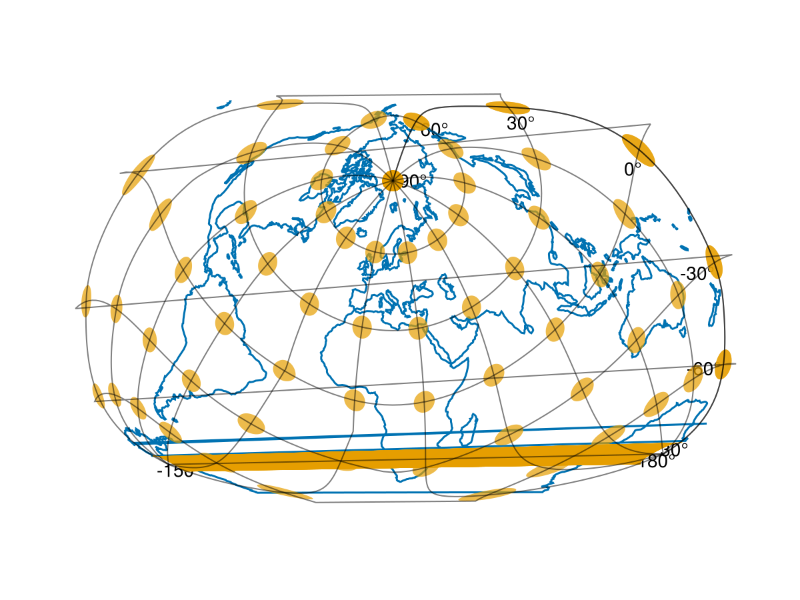
Tissot's indicatrix is a way to characterize local distortions in a map projection (see the Wikipedia article).
It is traditionally constructed by differentiating the projection. However, we can implement a similar indicatrix in a more straightforward way, by simply projecting circles formed on the ellipsoidal Earth onto a map.
Here' we'll show how you can do this for a few projections.
using Proj, GeoMakie, CairoMakie
import GeometryBasics: Point2d
import GeometryOps as GO
First, we define a function that gets a geodesic circle around some given point:
function geodesic_circle(origin::PointType, radius::Real, npoints = 100; geodesic = Proj.geod_geodesic(6378137, 1/298.257223563)) where PointType <: Point2
points = [PointType(Proj.geod_direct(geodesic, origin[2], origin[1], θ, radius)[[2, 1]]) for θ in LinRange(0, 360, npoints)]
if points[end] != points[begin]
points[end] = points[begin]
end
return points
end
poly(geodesic_circle(Point2f(0, 65), 100_000); axis = (; aspect = DataAspect()))
Note the curvature of the polygon - this is because it's the locus of points which are radius
away from origin
on the ellipsoidal Earth, not the flat Earth!
Now, we can create a proper Tissot map. Let's examine the Bertin 1953 projection.
f, a, p = lines(GeoMakie.coastlines(); axis = (; type = GeoAxis, dest = "+proj=bertin1953"))
f
Now, we cn add the Tissot polygons:
lons = LinRange(-180, 180, 13)
lats = LinRange(-90, 90, 7)
circle_polys = [GO.GI.Polygon([geodesic_circle(Point2d(lon, lat), 500_000, 50)]) for lon in lons, lat in lats] |> vec
91-element Vector{GeoInterface.Wrappers.Polygon{false, false, Vector{GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}}, Nothing, Nothing}}:
GeoInterface.Wrappers.Polygon{false, false, Vector{GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}}, Nothing, Nothing}(GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}[GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}(GeometryBasics.Point{2, Float64}[[-180.0, -85.52339101263448], [-172.6530612244898, -85.52339101263448], [-165.3061224489796, -85.52339101263448], [-157.9591836734694, -85.52339101263448], [-150.6122448979592, -85.52339101263448], [-143.26530612244898, -85.52339101263448], [-135.91836734693877, -85.52339101263448], [-128.57142857142856, -85.52339101263448], [-121.22448979591836, -85.52339101263448], [-113.87755102040816, -85.52339101263448] … [113.87755102040819, -85.52339101263448], [121.22448979591837, -85.52339101263448], [128.57142857142856, -85.52339101263448], [135.9183673469388, -85.52339101263448], [143.26530612244898, -85.52339101263448], [150.61224489795921, -85.52339101263448], [157.9591836734694, -85.52339101263448], [165.30612244897958, -85.52339101263448], [172.65306122448976, -85.52339101263448], [-180.0, -85.52339101263448]], nothing, nothing)], nothing, nothing)
GeoInterface.Wrappers.Polygon{false, false, Vector{GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}}, Nothing, Nothing}(GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}[GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}(GeometryBasics.Point{2, Float64}[[-150.0, -85.52339101263448], [-142.6530612244898, -85.52339101263448], [-135.3061224489796, -85.52339101263448], [-127.9591836734694, -85.52339101263448], [-120.61224489795919, -85.52339101263448], [-113.26530612244898, -85.52339101263448], [-105.91836734693877, -85.52339101263448], [-98.57142857142857, -85.52339101263448], [-91.22448979591836, -85.52339101263448], [-83.87755102040816, -85.52339101263448] … [143.8775510204082, -85.52339101263448], [151.22448979591837, -85.52339101263448], [158.57142857142856, -85.52339101263448], [165.9183673469388, -85.52339101263448], [173.26530612244898, -85.52339101263448], [-179.38775510204079, -85.52339101263448], [-172.0408163265306, -85.52339101263448], [-164.69387755102042, -85.52339101263448], [-157.34693877551024, -85.52339101263448], [-150.0, -85.52339101263448]], nothing, nothing)], nothing, nothing)
GeoInterface.Wrappers.Polygon{false, false, Vector{GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}}, Nothing, Nothing}(GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}[GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}(GeometryBasics.Point{2, Float64}[[-120.0, -85.52339101263448], [-112.65306122448979, -85.52339101263448], [-105.3061224489796, -85.52339101263448], [-97.9591836734694, -85.52339101263448], [-90.61224489795919, -85.52339101263448], [-83.26530612244898, -85.52339101263448], [-75.91836734693877, -85.52339101263448], [-68.57142857142857, -85.52339101263448], [-61.22448979591836, -85.52339101263448], [-53.87755102040816, -85.52339101263448] … [173.8775510204082, -85.52339101263448], [-178.77551020408163, -85.52339101263448], [-171.42857142857144, -85.52339101263448], [-164.0816326530612, -85.52339101263448], [-156.73469387755102, -85.52339101263448], [-149.38775510204079, -85.52339101263448], [-142.0408163265306, -85.52339101263448], [-134.69387755102042, -85.52339101263448], [-127.34693877551024, -85.52339101263448], [-120.0, -85.52339101263448]], nothing, nothing)], nothing, nothing)
GeoInterface.Wrappers.Polygon{false, false, Vector{GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}}, Nothing, Nothing}(GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}[GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}(GeometryBasics.Point{2, Float64}[[-90.0, -85.52339101263448], [-82.65306122448979, -85.52339101263448], [-75.3061224489796, -85.52339101263448], [-67.9591836734694, -85.52339101263448], [-60.61224489795919, -85.52339101263448], [-53.265306122448976, -85.52339101263448], [-45.91836734693877, -85.52339101263448], [-38.57142857142857, -85.52339101263448], [-31.22448979591836, -85.52339101263448], [-23.877551020408163, -85.52339101263448] … [-156.1224489795918, -85.52339101263448], [-148.77551020408163, -85.52339101263448], [-141.42857142857144, -85.52339101263448], [-134.0816326530612, -85.52339101263448], [-126.73469387755102, -85.52339101263448], [-119.38775510204079, -85.52339101263448], [-112.0408163265306, -85.52339101263448], [-104.69387755102042, -85.52339101263448], [-97.34693877551024, -85.52339101263448], [-90.0, -85.52339101263448]], nothing, nothing)], nothing, nothing)
GeoInterface.Wrappers.Polygon{false, false, Vector{GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}}, Nothing, Nothing}(GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}[GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}(GeometryBasics.Point{2, Float64}[[-60.000000000000014, -85.52339101263448], [-52.65306122448981, -85.52339101263448], [-45.30612244897961, -85.52339101263448], [-37.959183673469404, -85.52339101263448], [-30.6122448979592, -85.52339101263448], [-23.26530612244899, -85.52339101263448], [-15.918367346938787, -85.52339101263448], [-8.571428571428584, -85.52339101263448], [-1.2244897959183731, -85.52339101263448], [6.122448979591823, -85.52339101263448] … [-126.12244897959182, -85.52339101263448], [-118.77551020408166, -85.52339101263448], [-111.42857142857144, -85.52339101263448], [-104.0816326530612, -85.52339101263448], [-96.73469387755104, -85.52339101263448], [-89.3877551020408, -85.52339101263448], [-82.04081632653062, -85.52339101263448], [-74.69387755102044, -85.52339101263448], [-67.34693877551025, -85.52339101263448], [-60.000000000000014, -85.52339101263448]], nothing, nothing)], nothing, nothing)
GeoInterface.Wrappers.Polygon{false, false, Vector{GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}}, Nothing, Nothing}(GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}[GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}(GeometryBasics.Point{2, Float64}[[-29.999999999999986, -85.52339101263448], [-22.653061224489782, -85.52339101263448], [-15.306122448979583, -85.52339101263448], [-7.959183673469376, -85.52339101263448], [-0.6122448979591724, -85.52339101263448], [6.734693877551038, -85.52339101263448], [14.081632653061241, -85.52339101263448], [21.428571428571445, -85.52339101263448], [28.775510204081655, -85.52339101263448], [36.12244897959185, -85.52339101263448] … [-96.1224489795918, -85.52339101263448], [-88.77551020408163, -85.52339101263448], [-81.42857142857142, -85.52339101263448], [-74.08163265306118, -85.52339101263448], [-66.73469387755101, -85.52339101263448], [-59.38775510204077, -85.52339101263448], [-52.04081632653059, -85.52339101263448], [-44.69387755102041, -85.52339101263448], [-37.346938775510225, -85.52339101263448], [-29.999999999999986, -85.52339101263448]], nothing, nothing)], nothing, nothing)
GeoInterface.Wrappers.Polygon{false, false, Vector{GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}}, Nothing, Nothing}(GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}[GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}(GeometryBasics.Point{2, Float64}[[0.0, -85.52339101263448], [7.346938775510203, -85.52339101263448], [14.693877551020403, -85.52339101263448], [22.04081632653061, -85.52339101263448], [29.387755102040813, -85.52339101263448], [36.734693877551024, -85.52339101263448], [44.08163265306123, -85.52339101263448], [51.42857142857143, -85.52339101263448], [58.77551020408164, -85.52339101263448], [66.12244897959184, -85.52339101263448] … [-66.12244897959181, -85.52339101263448], [-58.77551020408164, -85.52339101263448], [-51.42857142857144, -85.52339101263448], [-44.0816326530612, -85.52339101263448], [-36.734693877551024, -85.52339101263448], [-29.38775510204078, -85.52339101263448], [-22.040816326530603, -85.52339101263448], [-14.69387755102042, -85.52339101263448], [-7.346938775510239, -85.52339101263448], [0.0, -85.52339101263448]], nothing, nothing)], nothing, nothing)
GeoInterface.Wrappers.Polygon{false, false, Vector{GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}}, Nothing, Nothing}(GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}[GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}(GeometryBasics.Point{2, Float64}[[30.0, -85.52339101263448], [37.3469387755102, -85.52339101263448], [44.69387755102041, -85.52339101263448], [52.04081632653061, -85.52339101263448], [59.38775510204081, -85.52339101263448], [66.73469387755102, -85.52339101263448], [74.08163265306123, -85.52339101263448], [81.42857142857143, -85.52339101263448], [88.77551020408164, -85.52339101263448], [96.12244897959184, -85.52339101263448] … [-36.12244897959181, -85.52339101263448], [-28.77551020408164, -85.52339101263448], [-21.428571428571438, -85.52339101263448], [-14.081632653061199, -85.52339101263448], [-6.734693877551024, -85.52339101263448], [0.6122448979592185, -85.52339101263448], [7.959183673469397, -85.52339101263448], [15.30612244897958, -85.52339101263448], [22.65306122448976, -85.52339101263448], [30.0, -85.52339101263448]], nothing, nothing)], nothing, nothing)
GeoInterface.Wrappers.Polygon{false, false, Vector{GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}}, Nothing, Nothing}(GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}[GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}(GeometryBasics.Point{2, Float64}[[59.99999999999999, -85.52339101263448], [67.3469387755102, -85.52339101263448], [74.69387755102039, -85.52339101263448], [82.0408163265306, -85.52339101263448], [89.38775510204081, -85.52339101263448], [96.73469387755102, -85.52339101263448], [104.08163265306122, -85.52339101263448], [111.42857142857142, -85.52339101263448], [118.77551020408163, -85.52339101263448], [126.12244897959184, -85.52339101263448] … [-6.122448979591816, -85.52339101263448], [1.2244897959183518, -85.52339101263448], [8.571428571428555, -85.52339101263448], [15.918367346938794, -85.52339101263448], [23.26530612244897, -85.52339101263448], [30.61224489795921, -85.52339101263448], [37.95918367346939, -85.52339101263448], [45.30612244897957, -85.52339101263448], [52.653061224489754, -85.52339101263448], [59.99999999999999, -85.52339101263448]], nothing, nothing)], nothing, nothing)
GeoInterface.Wrappers.Polygon{false, false, Vector{GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}}, Nothing, Nothing}(GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}[GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}(GeometryBasics.Point{2, Float64}[[90.0, -85.52339101263448], [97.34693877551021, -85.52339101263448], [104.6938775510204, -85.52339101263448], [112.0408163265306, -85.52339101263448], [119.38775510204081, -85.52339101263448], [126.73469387755102, -85.52339101263448], [134.08163265306123, -85.52339101263448], [141.42857142857144, -85.52339101263448], [148.77551020408163, -85.52339101263448], [156.12244897959184, -85.52339101263448] … [23.87755102040819, -85.52339101263448], [31.22448979591836, -85.52339101263448], [38.57142857142856, -85.52339101263448], [45.9183673469388, -85.52339101263448], [53.265306122448976, -85.52339101263448], [60.612244897959215, -85.52339101263448], [67.9591836734694, -85.52339101263448], [75.30612244897958, -85.52339101263448], [82.65306122448976, -85.52339101263448], [90.0, -85.52339101263448]], nothing, nothing)], nothing, nothing)
⋮
GeoInterface.Wrappers.Polygon{false, false, Vector{GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}}, Nothing, Nothing}(GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}[GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}(GeometryBasics.Point{2, Float64}[[119.99999999999999, 85.52339101263448], [112.65306122448978, 85.52339101263448], [105.3061224489796, 85.52339101263448], [97.95918367346938, 85.52339101263448], [90.61224489795917, 85.52339101263448], [83.26530612244896, 85.52339101263448], [75.91836734693875, 85.52339101263448], [68.57142857142854, 85.52339101263448], [61.22448979591836, 85.52339101263448], [53.87755102040815, 85.52339101263448] … [-173.8775510204082, 85.52339101263448], [178.77551020408163, 85.52339101263448], [171.42857142857144, 85.52339101263448], [164.0816326530612, 85.52339101263448], [156.73469387755102, 85.52339101263448], [149.38775510204079, 85.52339101263448], [142.0408163265306, 85.52339101263448], [134.69387755102036, 85.52339101263448], [127.34693877551024, 85.52339101263448], [119.99999999999999, 85.52339101263448]], nothing, nothing)], nothing, nothing)
GeoInterface.Wrappers.Polygon{false, false, Vector{GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}}, Nothing, Nothing}(GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}[GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}(GeometryBasics.Point{2, Float64}[[150.0, 85.52339101263448], [142.65306122448982, 85.52339101263448], [135.30612244897964, 85.52339101263448], [127.95918367346941, 85.52339101263448], [120.6122448979592, 85.52339101263448], [113.26530612244899, 85.52339101263448], [105.91836734693878, 85.52339101263448], [98.57142857142857, 85.52339101263448], [91.22448979591839, 85.52339101263448], [83.87755102040818, 85.52339101263448] … [-143.8775510204082, 85.52339101263448], [-151.22448979591837, 85.52339101263448], [-158.57142857142856, 85.52339101263448], [-165.9183673469388, 85.52339101263448], [-173.26530612244898, 85.52339101263448], [179.38775510204079, 85.52339101263448], [172.0408163265306, 85.52339101263448], [164.69387755102042, 85.52339101263448], [157.34693877551024, 85.52339101263448], [150.0, 85.52339101263448]], nothing, nothing)], nothing, nothing)
GeoInterface.Wrappers.Polygon{false, false, Vector{GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}}, Nothing, Nothing}(GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}[GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}(GeometryBasics.Point{2, Float64}[[180.0, 85.52339101263448], [172.6530612244898, 85.52339101263448], [165.3061224489796, 85.52339101263448], [157.9591836734694, 85.52339101263448], [150.6122448979592, 85.52339101263448], [143.26530612244898, 85.52339101263448], [135.91836734693877, 85.52339101263448], [128.57142857142856, 85.52339101263448], [121.22448979591837, 85.52339101263448], [113.87755102040816, 85.52339101263448] … [-113.87755102040819, 85.52339101263448], [-121.22448979591837, 85.52339101263448], [-128.57142857142856, 85.52339101263448], [-135.9183673469388, 85.52339101263448], [-143.26530612244898, 85.52339101263448], [-150.61224489795921, 85.52339101263448], [-157.9591836734694, 85.52339101263448], [-165.3061224489796, 85.52339101263448], [-172.65306122448976, 85.52339101263448], [180.0, 85.52339101263448]], nothing, nothing)], nothing, nothing)
GeoInterface.Wrappers.Polygon{false, false, Vector{GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}}, Nothing, Nothing}(GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}[GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}(GeometryBasics.Point{2, Float64}[[-150.0, 85.52339101263448], [-157.3469387755102, 85.52339101263448], [-164.6938775510204, 85.52339101263448], [-172.0408163265306, 85.52339101263448], [-179.3877551020408, 85.52339101263448], [173.26530612244898, 85.52339101263448], [165.91836734693877, 85.52339101263448], [158.57142857142856, 85.52339101263448], [151.22448979591837, 85.52339101263448], [143.87755102040816, 85.52339101263448] … [-83.87755102040819, 85.52339101263448], [-91.22448979591837, 85.52339101263448], [-98.57142857142856, 85.52339101263448], [-105.9183673469388, 85.52339101263448], [-113.26530612244898, 85.52339101263448], [-120.61224489795921, 85.52339101263448], [-127.9591836734694, 85.52339101263448], [-135.3061224489796, 85.52339101263448], [-142.65306122448976, 85.52339101263448], [-150.0, 85.52339101263448]], nothing, nothing)], nothing, nothing)
GeoInterface.Wrappers.Polygon{false, false, Vector{GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}}, Nothing, Nothing}(GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}[GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}(GeometryBasics.Point{2, Float64}[[-120.0, 85.52339101263448], [-127.34693877551021, 85.52339101263448], [-134.6938775510204, 85.52339101263448], [-142.0408163265306, 85.52339101263448], [-149.3877551020408, 85.52339101263448], [-156.73469387755102, 85.52339101263448], [-164.08163265306123, 85.52339101263448], [-171.42857142857144, 85.52339101263448], [-178.77551020408163, 85.52339101263448], [173.87755102040816, 85.52339101263448] … [-53.8775510204082, 85.52339101263448], [-61.22448979591838, 85.52339101263448], [-68.57142857142856, 85.52339101263448], [-75.9183673469388, 85.52339101263448], [-83.26530612244898, 85.52339101263448], [-90.61224489795921, 85.52339101263448], [-97.9591836734694, 85.52339101263448], [-105.30612244897961, 85.52339101263448], [-112.65306122448976, 85.52339101263448], [-120.0, 85.52339101263448]], nothing, nothing)], nothing, nothing)
GeoInterface.Wrappers.Polygon{false, false, Vector{GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}}, Nothing, Nothing}(GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}[GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}(GeometryBasics.Point{2, Float64}[[-90.0, 85.52339101263448], [-97.34693877551024, 85.52339101263448], [-104.69387755102039, 85.52339101263448], [-112.0408163265306, 85.52339101263448], [-119.38775510204081, 85.52339101263448], [-126.73469387755102, 85.52339101263448], [-134.08163265306123, 85.52339101263448], [-141.42857142857144, 85.52339101263448], [-148.77551020408163, 85.52339101263448], [-156.12244897959184, 85.52339101263448] … [-23.87755102040819, 85.52339101263448], [-31.224489795918373, 85.52339101263448], [-38.571428571428555, 85.52339101263448], [-45.918367346938794, 85.52339101263448], [-53.265306122448976, 85.52339101263448], [-60.612244897959215, 85.52339101263448], [-67.9591836734694, 85.52339101263448], [-75.30612244897961, 85.52339101263448], [-82.65306122448976, 85.52339101263448], [-90.0, 85.52339101263448]], nothing, nothing)], nothing, nothing)
GeoInterface.Wrappers.Polygon{false, false, Vector{GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}}, Nothing, Nothing}(GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}[GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}(GeometryBasics.Point{2, Float64}[[-60.0, 85.52339101263448], [-67.34693877551024, 85.52339101263448], [-74.69387755102036, 85.52339101263448], [-82.0408163265306, 85.52339101263448], [-89.38775510204084, 85.52339101263448], [-96.73469387755102, 85.52339101263448], [-104.08163265306123, 85.52339101263448], [-111.42857142857144, 85.52339101263448], [-118.77551020408163, 85.52339101263448], [-126.12244897959184, 85.52339101263448] … [6.122448979591809, 85.52339101263448], [-1.2244897959183731, 85.52339101263448], [-8.571428571428555, 85.52339101263448], [-15.918367346938794, 85.52339101263448], [-23.265306122448976, 85.52339101263448], [-30.612244897959215, 85.52339101263448], [-37.9591836734694, 85.52339101263448], [-45.30612244897961, 85.52339101263448], [-52.65306122448976, 85.52339101263448], [-60.0, 85.52339101263448]], nothing, nothing)], nothing, nothing)
GeoInterface.Wrappers.Polygon{false, false, Vector{GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}}, Nothing, Nothing}(GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}[GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}(GeometryBasics.Point{2, Float64}[[-30.0, 85.52339101263448], [-37.34693877551024, 85.52339101263448], [-44.693877551020364, 85.52339101263448], [-52.0408163265306, 85.52339101263448], [-59.38775510204084, 85.52339101263448], [-66.73469387755102, 85.52339101263448], [-74.0816326530612, 85.52339101263448], [-81.42857142857144, 85.52339101263448], [-88.77551020408163, 85.52339101263448], [-96.12244897959181, 85.52339101263448] … [36.12244897959181, 85.52339101263448], [28.775510204081627, 85.52339101263448], [21.428571428571445, 85.52339101263448], [14.081632653061206, 85.52339101263448], [6.734693877551024, 85.52339101263448], [-0.612244897959215, 85.52339101263448], [-7.959183673469397, 85.52339101263448], [-15.306122448979607, 85.52339101263448], [-22.65306122448976, 85.52339101263448], [-30.0, 85.52339101263448]], nothing, nothing)], nothing, nothing)
GeoInterface.Wrappers.Polygon{false, false, Vector{GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}}, Nothing, Nothing}(GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}[GeoInterface.Wrappers.LinearRing{false, false, Vector{GeometryBasics.Point{2, Float64}}, Nothing, Nothing}(GeometryBasics.Point{2, Float64}[[0.0, 85.52339101263448], [-7.346938775510239, 85.52339101263448], [-14.693877551020364, 85.52339101263448], [-22.040816326530603, 85.52339101263448], [-29.387755102040842, 85.52339101263448], [-36.734693877551024, 85.52339101263448], [-44.081632653061206, 85.52339101263448], [-51.428571428571445, 85.52339101263448], [-58.77551020408163, 85.52339101263448], [-66.12244897959181, 85.52339101263448] … [66.12244897959181, 85.52339101263448], [58.77551020408163, 85.52339101263448], [51.428571428571445, 85.52339101263448], [44.081632653061206, 85.52339101263448], [36.734693877551024, 85.52339101263448], [29.387755102040785, 85.52339101263448], [22.040816326530603, 85.52339101263448], [14.693877551020393, 85.52339101263448], [7.346938775510239, 85.52339101263448], [0.0, 85.52339101263448]], nothing, nothing)], nothing, nothing)
circle_polys_cut = GO.cut.(circle_polys, (GO.GI.Line(Point2d[(0, -180), (0, 180)]),)) .|> GO.GI.MultiPolygon # hide
poly!(a, circle_polys; color = Makie.wong_colors(0.7)[2])
f
This page was generated using Literate.jl.